While working from home during the past few months, I have greatly missed being able to throw some prototypes through the super nice SparkFun production reflow oven.
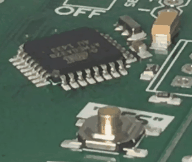
I really wanted a reflow oven in my garage, so after doing some research online, I decided to buy a cheap toaster oven and give this hack a try. Ultimately, I decided to use a standard hobby servo to automate the movement of the temperature knob like so:
Although slightly less elegant than some of the other methods out there, this servo control method works quite well. Additionally, it didn't require any modifications to the wiring of my toaster oven and was pretty darn cheap and simple to setup.
Research
I came across many blogs, websites and products dedicated to hacking toaster ovens into reflow ovens:
- SparkFun Reflow Toaster Tutorial (2006)
- SparkFun Reflow Toaster Controller (retired product from 2007)
- X-Toaster Article - choosing an oven
- CompuPhase Article - understanding reflow profiles
The most important things I learned were the following:
-
Get a toaster oven with these features (I purchased the Black & Decker TO3000G, which was $59 new):
- Analog dial control style
- Quartz elements (two above and two below if you can)
- A convection fan
- Small or medium-sized
-
A single thermocouple "floating" in the air near the PCB works fine. Note, a lot of the pro-end models have multiple temp sensors (at multiple points in the chamber and one touching the PCB), but I haven't found the need yet. The SparkFun Qwiic Thermocouple and braided thermocouple work great!
-
Opening the door seems to be the only way to get a good and fast cool down. Even the ~$4,000 pro machines have the drawer open automatically to achieve good "free air" cooling. I have yet to automate this step, but so far I love watching the solder flow. When I see my reflow time is up, I have been manually opening the door. If I find the need to automate this in the future, I'll most likely get another servo or stepper motor involved.
-
Most toaster ovens can't achieve an ideal ramp up rate of 1.5C/second, but that's okay. You can add a slower ramp up during your soak phase - a slow ramp up from 150-180. More on this later as we discuss the profile, the code and show some results.
Parts List
- Redboard Artemis
- SparkFun Qwiic Thermocouple Amplifier - MCP9600 (Screw Terminals)
- Thermocouple Type-K - Glass Braid Insulated (Bare Wire)
- Qwiic Cable - 100mm
- Servo - Hitec HS-422 (Standard Size)
- USB-C Wall Adapter - 5.1V, 3A (Black)
- Reversible USB A to C Cable - 2m
- Solder Paste - 50g (Lead Free)
- 3M Dual Lock (or servo tape)
- Control Rods - find at your local hobby or hardware shop
- Two large servo horns (you should get some with your servo, but may need to get a second one)
Manual testing
Before rigging up a servo to move the control knob, I first gathered parts and got my thermocouple readings working. Then the plan was to simply move the control knob manually (with my hand), and see if I could get a decent profile while watching the data plotter.
Following the profile suggestions in the CompuPhase article and reading what Wikipedea had, I decided to try for a profile represented by the blue line in the graphic above. The red represents the live readings from the thermocouple. Note: in all of my serial plotter graphics, the blue line is being plotted as a reference, and my controller is not analyzing the blue line in any way.
And so I tried again:
As you can see, I started taking notes to keep track of what was working and what was not. For my second attempt, I actually set the oven to "warm" for ten seconds before starting my plotter. The adjustments noted in the graphic were done by me physically moving the dial with my hand.
By my fourth manual test, I was pretty pleased with the results - not perfect by any means, but starting to look a bit like an actual profile:
Servo Hack
Now that I knew a decent profile could be achieved by simply moving the temp dial, it was time to slap a servo on this thing and automate the movements!
First things first, I ripped off that control knob. A flat head screw driver helped greatly to get it started. Next, I mounted the servo horn to the knob with a servo mount screw in the center hole of the servo horn.
Temp knob removed. | Mount with center point screw first. |
Then, I drilled out two more holes for a good secure mount. Note: I'd make sure to align the positioning mark (that little silver thing) directly down. This will ensure that the servo linkage and rotation can achieve the full 180 degrees of motion.
Mounted servo horn with center screw. | Horn mounting complete. |
Next was actually mounting the servo to the oven. I had a scrap piece of wood that fit the job exactly, but anything that can extend the servo out past the face of the oven should work. I opted to use dual-lock hook and loop, but servo tape or double-sided foam tape would have worked too. Make sure to align properly both along the center point of the servo horns and the depth away from the oven.
Center point alignment. | Depth alignement. |
Finally, the last step was to link the two control horns. There are many ways to do this with various linkage hardware, but the simplest is to use bare control rods and add "Z-bends" at specific points. I first tried this with a single control rod, but it was much smoother with two!
Z-bend | Dual control rod hooked up |
The Code
The source code for the project can be downloaded from the GitHub repository here:
Using Example 1 from the Qwiic Thermocouple Arduino Library and a basic servo control example, I was able to piece together a sketch that accomplishes a pretty nice reflow profile.
Before diving into the code, I'd like to highlight the fact that this project does not use PID control to try and follow a set profile. I first proved out that manually moving the temp knob by hand could create a decent reflow profile. By watching the temperature and keeping track of time, my Arduino can determine the zone (preheat, soak, ramp up, reflow, cooldown). Once you know the zone, you can simply set servo to predetermined positions (or toggle on/off as in soak) to hold a close enough temperature or ramp up.
My plan was:
- Set full power
- Wait to see 140C, kill power, enter soak zone
- During soak, toggle power on/off to climb from 150C to 180C
- Once soak time is complete, turn on full power (enter ramp-up zone)
- During ramp-up, wait to see reflow temp (220C) (enter reflow zone)
- During reflow, toggle servo on/off to hold temp
- Once reflow time is complete, turn servo to "off," indicating user should open door
My main loop simply uses a "for loop" to get through the zones, then when it's done it stops with a "while(1)". It is updateServoPos()
that actually determines the zone and updates a global variable.
void loop() {
for (int i = 0 ; i <= 4 ; i++) // cycle through all zones - 0 through 4.
{
int tempDelta = abs(profileTemp[i + 1] - profileTemp[i]);
int timeDelta = abs(profileTimeStamp[i + 1] - profileTimeStamp[i]);
float rampIncrementVal = float(tempDelta) / float(timeDelta);
currentTargetTemp = profileTemp[i];
// Print data to serial for good plotter view in real time.
// Print target (for visual reference only), currentTemp, ovenSetting (aka servo pos), zone
for (int j = 0 ; j < (timeDelta - 1); j++)
{
currentTargetTemp += rampIncrementVal;
Serial.print(currentTargetTemp, 2);
Serial.print(",");
if (tempSensor.available())
{
currentTemp = tempSensor.getThermocoupleTemp();
}
else {
currentTemp = 0; // error
}
Serial.print(currentTemp);
Serial.print(",");
updateServoPos(); // Note, this will increment the zone when specific times or temps are reached.
Serial.print(ovenSetting);
Serial.print(",");
Serial.println(zone * 20);
delay(1000);
totalTime++;
}
}
// monitor actual temps during cooldown
for (int i = 0 ; i < COOL_DOWN_SECONDS ; i++)
{
currentTargetTemp = 0;
Serial.print(currentTargetTemp, 2);
Serial.print(",");
if (tempSensor.available())
{
currentTemp = tempSensor.getThermocoupleTemp();
}
else {
currentTemp = 0; // error
}
Serial.print(currentTemp);
Serial.print(",");
updateServoPos();
Serial.print(ovenSetting);
Serial.print(",");
Serial.println(zone * 20);
delay(1000);
}
while (1); // end
}
The following function, updateServoPos()
, is where the temperature and totalTime is checked to determine the zone we are currently in, and ultimately what servo positions are desired.
void updateServoPos()
{
if ((zone == ZONE_preheat) && (currentTemp > 140)) // done with preheat, move onto soak
{
zone = ZONE_soak;
}
else if ((zone == ZONE_soak) && (soakSeconds > SOAK_TIME)) // done with soak, move onto ramp
{
zone = ZONE_ramp;
}
else if ((zone == ZONE_ramp) && (currentTemp > 215)) // done with ramp move onto reflow
{
zone = ZONE_reflow;
}
else if ((zone == ZONE_reflow) && (reflowSeconds > 30))
{
zone = ZONE_cool;
}
switch (zone) {
case ZONE_preheat:
setServo(41);
break;
case ZONE_soak:
soakSeconds++;
if ((soakSeconds % 15) == 0) // every 15 seconds toggle
{
soakState = !soakState;
}
if (soakState)
{
setServo(100);
}
else {
setServo(0);
}
break;
case ZONE_ramp:
setServo(100);
break;
case ZONE_reflow:
reflowSeconds++;
if ((reflowSeconds > 5) && (reflowSeconds < 10)) // from 5-10 seconds drop to off
{
setServo(0);
}
else {
setServo(100);
}
break;
case ZONE_cool:
setServo(0);
break;
}
}
Some of these settings would surely need to be tweaked when using a different oven, thermocouple and servo setup. Slight variations in the servo hardware hookups will most likely require different servo positions settings to get the desired position on the temp knob.
Also, the position of the thermocouple can affect its readings, so each setup will most likely require different temperature trigger values (i.e. in the servoUpdatePos() function, you may need to adjust the if statements that determine the zone).
Last, paste and component size must be considered. After reflowing a few panels, I saw that the SAC305 paste I was using actually started reflowing closer to 220C, so I made that my target max reflow temp. And if I had huge components, these can act as heatsinks and may require a longer soak and/or higher peak reflow temp.
Results
The above screenshot is from my latest achieved profile using servo control. The different colored lines represent the following:
- Blue: Generic reference profile
- Red: Temp readings showing actual profile in the oven
- Yellow: Zones, stepping up through preheat, soak, ramp-up, reflow, cooldown
- Green: Servo position, represented by 0-100 percent power
Although this last profile screenshot is the result of almost 20 test runs and 10 actual board panels reflowing, I would like to mention that even with some slight variation between profiles, all of my panels came out beautifully. A good paste job and proper placement of parts can greatly help your initial yield, but I even had a few sloppy paste jobs and they came out fine!
Conclusion
My complete setup in the garage. | Panel in the oven. |
If you're interested in reflowing some electronics using a toaster oven, it can be done using a simple Arduino, servo and thermocouple. It does take a little time to do some test runs and make slight adjustments to the provided Arduino sketch, but the results are pretty great! I currently have to watch the oven to manually open the door when it's done, but this could be automated. Plus, while one panel was in the oven, I simply started hand placing parts on the next panel and so no time was really lost.
If you would like to give this a try and have any questions, please reach out in the comment below or at the GitHub project repository.
Also, if you have any experience with solder reflow profiles, I'd love to hear your thoughts in the comments below.
Thanks for reading and cheers to some good DIY electronics SMD assembly!
Resources
- Project Github Repository (contains Arduino Source Code)
- Thermocouple Hookup Guide
- Servo Control Tutorial
- X-Toaster Article - choosing an oven
- CompuPhase Article - understanding reflow profiles
No comments:
Post a Comment